In case of I don’t see you. Good afternoon, good evening and good night.
在xml预览中查看RecyclerView布局
在功能开发的前期,我们做好布局后,希望可以在IDE的xml预览里查看效果。在使用到RecyclerView时,看到的往往是item 0、item 1、item 2这样的占位文字,如下左图所示。如果我们想查看设计好的item布局(如下右),应当如何做呢?
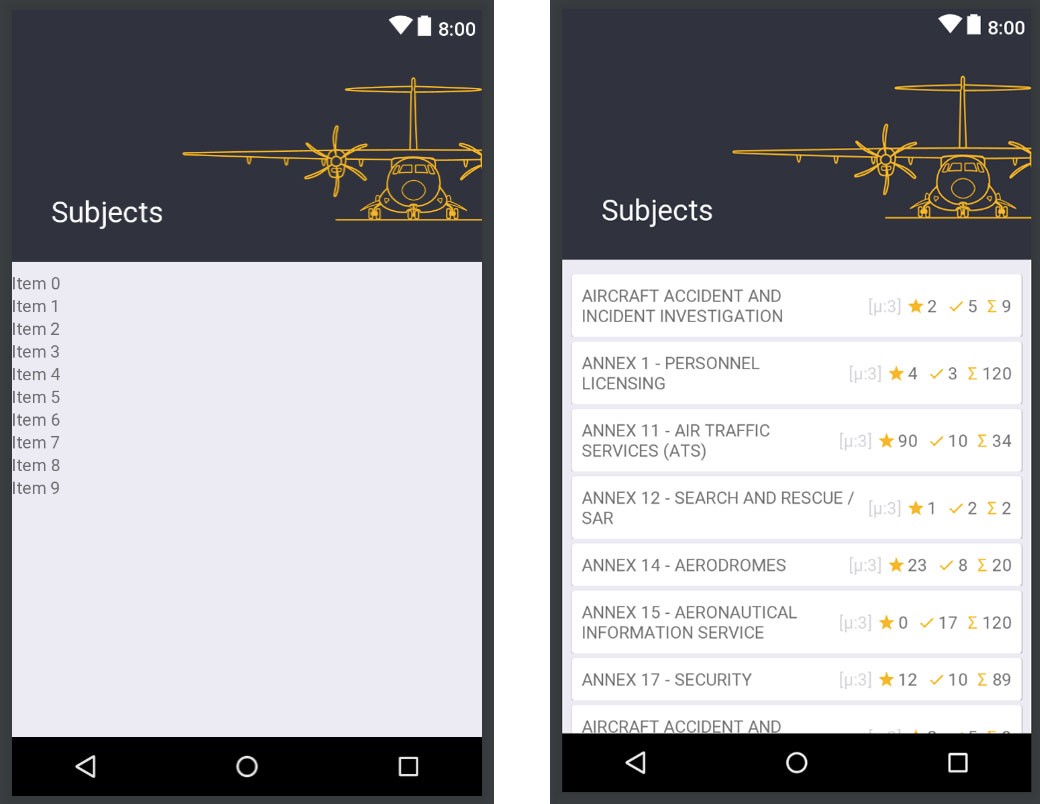
可以使用tools的命名空间。
tools
namespace enables design-time features (such as which layout to show in a fragment) or compile-time behaviors (such as which shrinking mode to apply to your XML resources) It is really powerful feature that is developing and allows you not compile code every time to see changes.
1 | <!-- 样例代码 --> |
Uri对象的基本操作
不属于新知识,做网络请求开发时经常会遇到,以前每次碰到时都是现查的,不知道是不是年纪大了记忆力下降的缘故 :-( 这里整理作为备忘。
Uri是不可变对象,构建出来后即不可修改。
Uri采用构建器模式进行构建。
初始化一个Uri.Builder对象:Uri.parse(SAMPLE_URL).buildUpon()
,如下例
1 | val uriBuilder = Uri.parse("https://www.google.com").buildUpon() |
进行UTF8编码:encode(String s)
增加参数:appendQueryParameter("key", "value")
分开设置协议和主机:scheme("https")
,authority("www.google.com")
举例说明,如果想要构建如下的URL地址
1 | https://www.myawesomesite.com/turtles/types?type=1&sort=relevance#section-name |
则使用以下代码实现
1 | Uri.Builder builder = new Uri.Builder(); |
参考
- https://developer.android.com/reference/android/net/Uri
- https://developer.android.com/reference/android/net/Uri.Builder
轻松创建MainDispatcher与Deferred
构建主线程调度器
Dispatcher/调度器是协程上下文里很重要的一个元素,它决定了代码运行在哪个线程中。尤其是做Android开发时,经常需要把UI操作发送给主线程进行,像ViewModel就为我们提供了MainDispatcher。
如果当前没有MainDispatcher,需要手动创建时,要怎么方便地进行构建呢?Kotlin在Handler
类上新增了一个扩展函数asCoroutineDispatcher()
,用法如下
1 | val mainDispatcher = Handler(Looper.getMainLooper()).asCoroutineDispatcher() |
只要通过MainLooper就可以构建出主线程调度器了,是不是很方便。
构建即时Deferred对象
Deferred是一种泛型,在协程中用于表示耗时操作结果,当我们需要取值时,通过Deferred.await()
即可。它有点类似Java中的Future
,表示一种“进行中的操作,即将返回结果”。
构建Deferred对象有两种方法,一种是通过async
的携程构建器,另一种是今天要介绍的方法,对于已知的值(比如 10),可以直接用CompletableDeferred(10)
来创建一个Deferred对象,你可以直接把它用在任何需要Deferred对象的地方,取值时则会直接返回10。
参考 https://stackoverflow.com/questions/53273361/how-to-return-deferred-with-the-instant-results